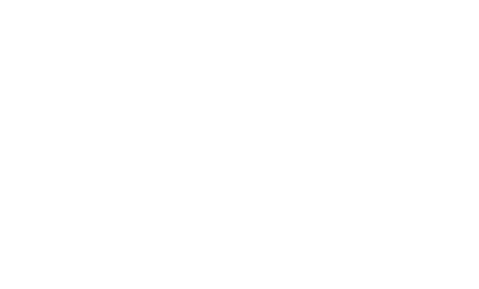
Foundation
Javascript
Foundation 4 streamlines the implementation of the Foundation plugins by combining them all into a single plugin under the $.fn.foundation()
Zepto/jQuery namespace.
Installation
Foundation JavaScript was designed to work with Zepto and jQuery right out of the gate. Zepto is not supported by all browsers, so as suggested in the Zepto documentation, you should test for compatibility and load Zepto or jQuery as necessary.
Include Libraries
In the head
section of your page add Modernizr. Modernizr acts as a shim for HTML5 elements for older browsers as well as detection for mobile devices.
<script src="/js/custom.modernizr.js"></script>
Before the closing body
tag, insert the following JavaScript.
<!-- Check for Zepto support, load jQuery if necessary -->
<script>
document.write('<script src=/js/vendor/'
+ ('__proto__' in {} ? 'zepto' : 'jquery')
+ '.js><\/script>');
</script>
Then include either foundation.min.js
, which includes Foundation Core and all JavaScript plugins or you can load them individually. The minified version is not available in the Compass version.
<script src="/js/foundation.min.js"></script>
<!-- or individually -->
<script src="/js/foundation.js"></script>
<script src="/js/foundation.alerts.js"></script>
<!-- ... -->
<script src="/js/foundation.dropdown.js"></script>
<script src="/js/foundation.section.js"></script>
Initialize Foundation
After you have included the Foundation JavaScript, just add a simple call to initialize all plugins on your page.
It is recommended that you initialize Foundation at the end of the page <body>
.
<script>
$(document).foundation();
</script>
Note: We include tested versions of jQuery and Zepto in the Foundation repo to get you started quickly. If you want to build your own version of Zepto, Foundation employs the event, fx_methods, assets, data, selector, and stack modules.
Configuration
Foundation accepts a myriad of options for initialization. By default, calling $('#scope').foundation();
will initialize all available plugins on the page. Alternatively, you can pass individual plugins along with configuration options and a callback. This will allow you to select specific plugins to start on your page, even if their source is included. You can take a look at the call here:
$(document).foundation('section', {deep_linking: true}, function (response) {
console.log(response.errors);
});
The call will initialize the Section plugin on the page with deep linking enabled and pass back a response object with any errors that may have occurred.
You can initialize multiple libraries within the same call as well. Here are a few ways this can be done:
$(document).foundation('section dropdown alerts', {callback: myCallbackFunction}, function (response) {
console.log(response.errors);
});
// alternative, no configuration
$(document).foundation('section dropdown alerts', function (response) {
console.log(response.errors);
});
// alternative, all plugins, only callback
$(document).foundation(function (response) {
console.log(response.errors);
});
// alternative, only plugin, no config or callback
$(document).foundation('section');
Keep in mind, if you initialize multiple plugins at one time and pass a configuration object that object will get passed to all plugins that were initialized. So in the first example: section, dropdown, and alerts will all get passed the myCallbackFunction
on initialization.
What if you wanted to pass the myCallbackFunction
only to Section and still initialize the other plugins as well? You could do the following.
$(document)
.foundation('dropdown alerts')
.foundation('section', {callback: myCallbackFunction});
Calling Internal Methods
Foundation 4 JavaScript allows you call internal plugin methods by passing the method name as the second argument. This is necessary for plugins like Joyride, since they are not initialized on page load by default.
This will fire the start
method on Joyride:
$(document).foundation('joyride', 'start');
Unbind Events
All plugins can be unbound from the DOM by firing the off
event either on that plugin or globally to unbind all Foundation events.
$(document).foundation('tooltips', 'off');
// or globally
$(document).foundation('off');